Angular questions - PART 3
Getting started
This is my personal favorite article on the most Angular interview questions. I am writing about some more “advanced” topics and familiarizing yourself further on that is definitely going to be a game changer!
Let’s check what we are going to talk about:
1. What is Hydration
2. Unit testing
3. ng-content
4. What is a singleton in Angular
5. Design patterns in Angular
6. Structuring application
7. Services
8. Advantages of Angular
9. What is a MVVM architecture
10. Angular inbuilt pipes
11. Change detection
Let’s start with the first one.
What is a Hydration
First thing first - it is connected to Server Side Rendering.
Hydration is the process that restores the server side rendered application on the client.
One main feature is reusing the server rendered DOM structure, avoiding the extra work to re-create the DOM nodes. Angular tries to match the existing DOM elements at runtime and reuses DOM nodes when possible.
This is a huge performance booster!
In order to use it, Angular Universal is needed and it has to be bootstrapped in the following way
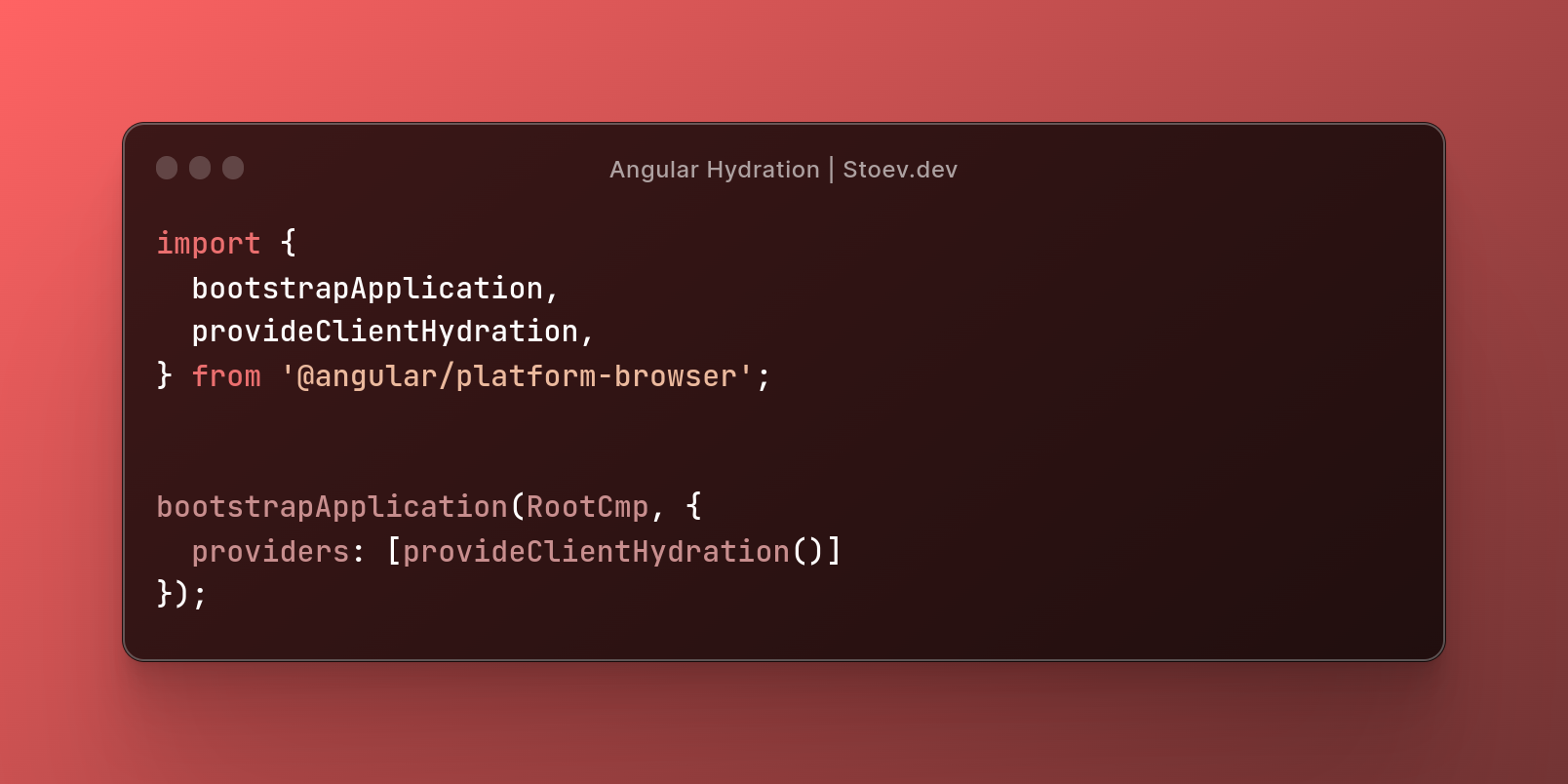
One thing to keep in mind.
The HTML produced by the server side rendering, must not be altered between the server and the client.
If mismatch is found, the hydration process will encounter problems attempting to match it up.
It’s a common problem with manipulating the DOM with native APIs such as innerHTML, appendChild and so on.
If you need that, you can skip the hydration for the component.
it is done with ngSkipHydration
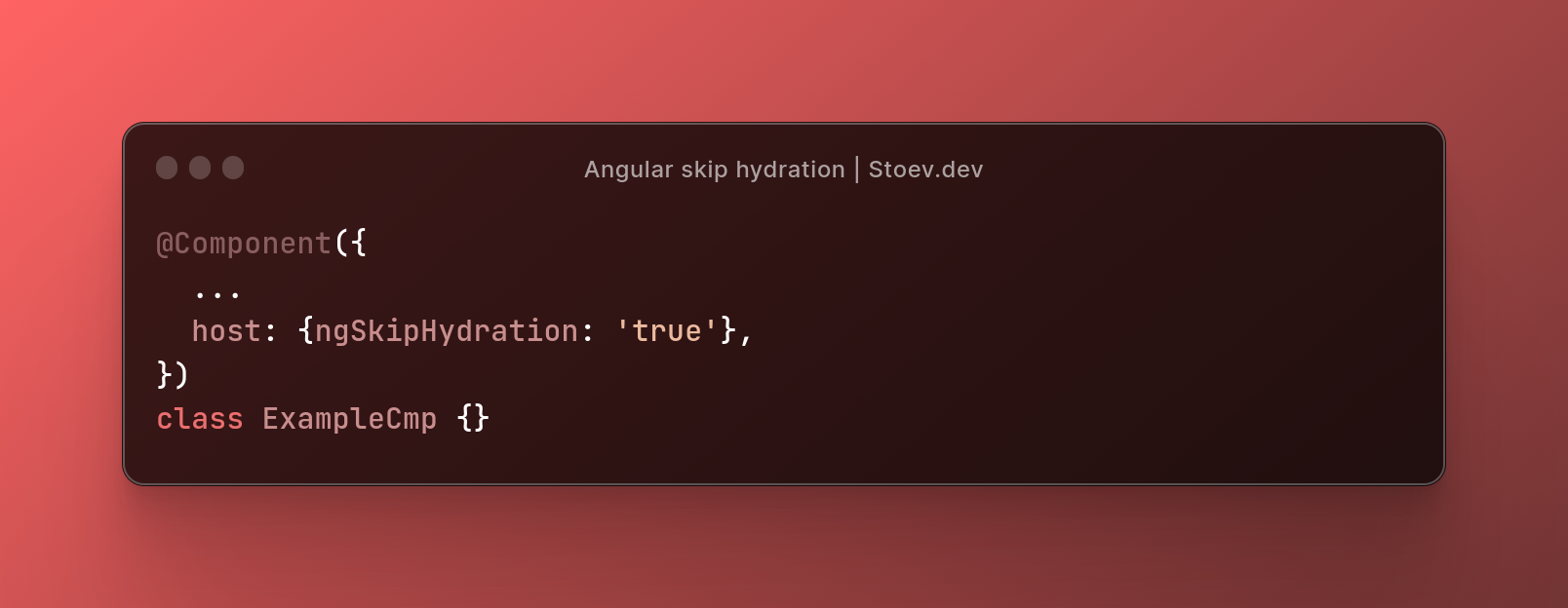
Another thing to mention is that hydration is dependent on zone.js and currently as of writing this article, there is no way to noop zone.js.
Unit testing in Angular
This is the process of testing isolated pieces of your code. This provides the advantage of catching and preventing bugs in the long term.
What does Angular use for testing?
Well, you can use anything and set up anything you like, but by default, Angular uses Jasmine and Karma.
Jasmine is a behavior driven development framework for testing JavaScript code.
It is a very popular and mature framework.
Karma is the task runner for the tests. It executes the Jasmine code and runs it either in the browser or in a headless browser environment.
The configuration of Jasmine and Karma is done by the Angular CLI.
It is a good practice to include testing in the CI!
ng-content
It is one of the best features of Angular, in my opinion.
This is a pattern for content projection, in which a content is inserted ( or projected ) inside another component.
Custom attributes applied to ng-content are ignored! It is merely a placeholder that doesn’t produce a DOM element by itself.
There are two main implementations
1. Single slot content projection
2. Multi slot content projection
Single-slot content projection
The most basic one, but it could get the job done in most of the cases.
using the <ng-content> tag, it is replaced with another component.
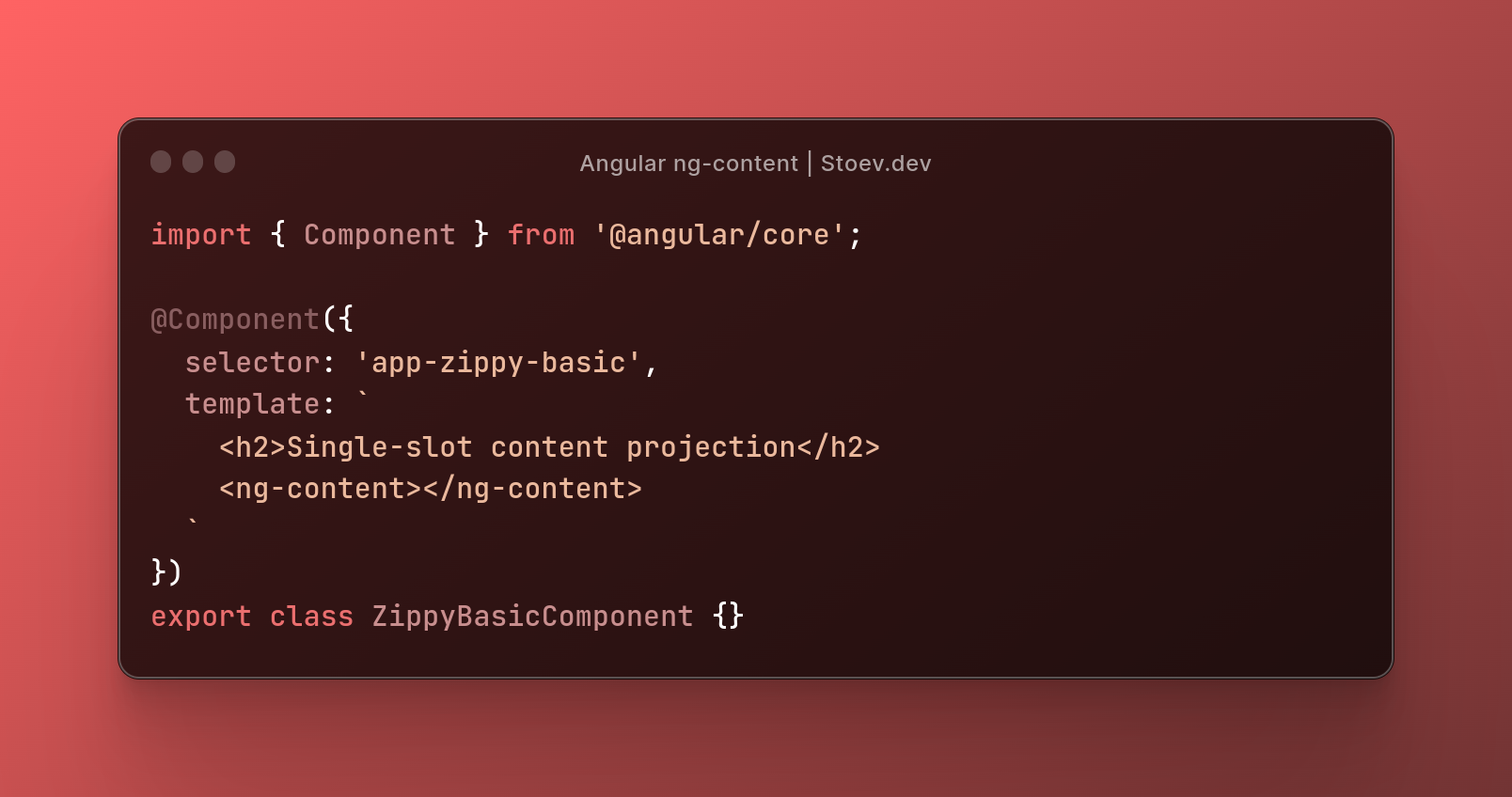
Multi-slot content projection
This is similar to the previous one, but it can have multiple slots for projections.
There is a selector that determines which content goes into the specific slot.
What is a singleton service in Angular
In Angular, we understand a service to be singleton, if only one instance exists in the application.
There are two ways to make a service singleton.
When created, in the @Injectable() we provideIn to root.
It is provided in the AppModule or in any module that is only imported by the AppModule.
Another way is to handle the import with a static forRoot() to separate providers from a module.
I have an article on the subject
- technology
- tutorial
- angular
Using forRoot() and forChild() in Angular
The article also provides additional information on how to prevent a module and a service to be reimported somewhere else in the application.
Design patterns in Angular
There are so many books on design patterns that simply cannot be summarized in one single response.
There are many patterns that could be implemented and all solutions to problems that occur during software development and design depends on the understanding and the capacity of the team.
There are few patterns that are rooted in the Angular practices.
We mentioned one of them above
The singleton pattern
This pattern is a design pattern that ensures a service is used from the same instance.
I introduced the idea to make a service singleton through the entire application. But there is a way to make it singleton in a specific module.
Just provide the service in the desired @NgModule({})
Facade Pattern
This is a structural design pattern that wraps a lot of complex logic and serves as a simplified interface to that logic.
It is very often used and implemented in Angular applications.
It's useful if components need to interact with a lot of different services or state management systems.
Hiding that in a FacadeService could decrease the complexity of the ts component. Everything is provided through the FacadeService and it’s easier to maintain.
Factory Pattern
A creational design pattern that adds an abstraction over common base behavior between multiple objects of a generic type.
Meaning you have a superclass that allows its subclasses to alter the type of the objects that will be created.
It is used often in Angular to create different components or services based on some condition or Input.
Observer Pattern
A software design pattern that a subject maintains a list of its dependents, called observers and notifies them automatically of any state changes.
It is often used for implementing event handling systems in event driven software.
In Angular it keeps components in sync.
You guess it right that Observables play a crucial role here.
Working declaratively and event driven is a perfect way to create performative, maintainable and reactive applications.
I have an article that talks more on the Observables and Events
- technology
- angular
- tutorial
How I work with Observables in Angular
Dependency Injection Pattern
This is a very important design pattern that is a backbone for Angular itself. Developing large and scalable applications without it is hard!
There are many Design patterns and knowing all of them and using them could be time consuming and hard.
I have a plan to write some articles on some specific Patterns so stay tuned and give me a follow if you are interested!
Structuring application
As of my experience I have seen two ways to structure an application.
I have heard that all Angular apps at some point start to look alike and I believe there is a good reason for that.
It forces you to write good and quality front end code!
Regarding the structure, it is feature based and type based.
Feature based Angular structure
The best one, in my opinion.
Every functionality is divided by logic and positioned in a separate feature folder. Inside you nest your services, dumb components, constant information and so on, but entirely related to the feature!
Everything else should go either in a shared module or a core module.
Check the following article for more information on how I do that structuring.
- tutorial
- technology
- angular
How I structure my Angular applications
Type based
This is a structure in which logic is divided by type and it is not separated by logic.
You have a folder for components, services, constant data and so on.
For small applications it is fine, but it can quickly become a mess for maintainability.
Services
Angular services are classes that are instantiated in the creation of the module and have a very specific purpose. To control data, manipulate information and organize business logic.
It can be used as a dependency and Injected in any component.
Services are encouraged to have only one purpose and serve specific logic.
It has to be providedIn the root or any other component in order to be used.
Advantages of Angular
I have worked with Angular and React in the past.
This is going to be a biased answer.
Angular is well more heavy and complete. It forces the devs to follow patterns, quality and it is made for enterprise and serious applications.
I have heard some full stack devs tell me that Angular is the front end that should be coded and I completely agree with that!
One of the advantages I find is:
1. Dependency Injection - controlling logic and state in big apps is easier
2. Difficult - some may think I am talking crap, but hear me out. Angular is harder and that means the devs have to learn more and more to get into it. The quality of devs is better.
3. Backed by Google - it means the long term game is secured.
4. Great resources - as I said, Angular devs tend to be more qualified and there are a lot of resources out there.
5. Easy testing - it comes out of the box.
6. It is actually reactive - in contrast to React… funny, right? React is actually not reactive?! WTF
7. It uses TypeScript out of the box - who is writing vanilla JS anyway these days.
Of course that doesn’t mean that there aren’t any cons and React is bad.
I mean.. it is bad, but if a good React devs set it up perfectly, it can make it as good as Angular.
I personally know great React devs, so I am not here to insult the dev community. It’s merely an observation I have made.
What is a MVVM architecture
This is an abbreviation of Model-View-ViewModel.
A software design pattern that is structured to separate program logic and user interface. The separation is as follows.
Model - This is the application’s data and business logic. It is in charge of retrieving and storing data from the database or API - interfaces, classes and types.
View - The user interface. It is in charge of displaying data to the user and recording user interaction - the HTML templates
ViewModel - a link and between the View and the Model - the components in Angular
Angular inbuilt pipes
Angular provides us with inbuilt pipes we can use to enhance the functionality of our components.
date
The date pipe is a pure pipe that transforms the format of the date.
The only local available with Angular is en-US
uppercase & lowercase
Transform the entire text to uppercase and lowercase respectfully.
currency
Transforms a number to a currency string.
It formats it according to locale rules.
decimal
Transforms a number into a string with a decimal point.
percent
Transforms a number to a percentage string, formatted according to locale rules.
Change detection
This is the process through which Angular checks for state changes.
Angular does that in specific cases. Triggering it can be done either manually or automatic.
It is very aggressive and every dev should try to optimize as much as possible.
It works by replacing the real event listeners in the browser APIs with a custom version that calls a callback that Angular utilizes for its change detection.
This is done thanks to zone.js
The default change detection strategy is performance heavy and aggressive. It checks every tree and it can quickly add up a lot of processes and worsen the performance.
To help that, onPush strategy is introduced that limits the way Angular runs its change detection mechanisms. It is run only in Input, event changes or async operations. It also skips the trees with onPush change detection if no triggerer ( event ) is present.
One thing people tend to forget is that change detection runs twice in development mode and only ones in production.
This is great for debugging purposes and sometimes can lead to unexpected behavior in development.
Pay attention to that!
Conclusion
This is not complete information, but a blueprint for what is important and what topics should be expanded and dived in.
Angular is too big and complex to understand for a few months or from a few articles.
As of the writing of this article, I have worked with it for around a couple of years and I still learn new stuff every few days.
It is a great choice for serious developers and it forces you to become a great programmer.
Happy coding and good luck!