Angular questions - PART 2
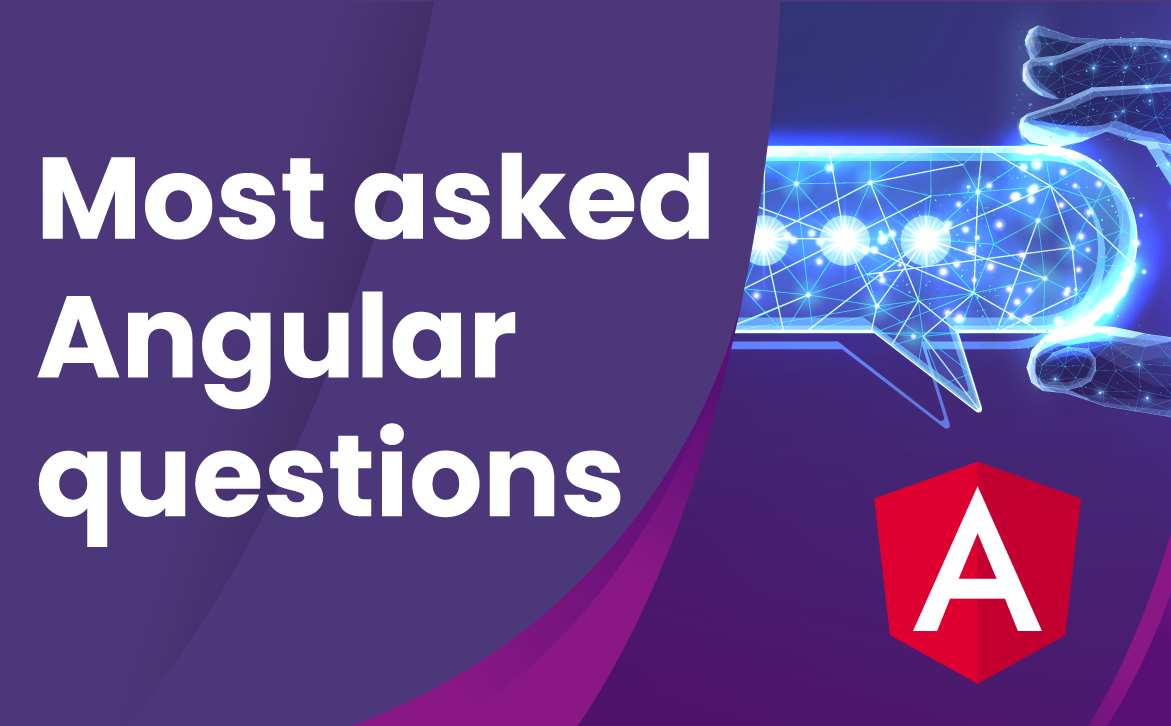
Getting started
This is the second of three articles on some of the most asked questions in Angular interviews. The list is nowhere near complete and every interview could be unique, depending on the interviewer.
But the knowledge and the common basics are usually the same.
Let’s write down what I am going to write about:
Error handling in Angular
Error handling in Angular has a few ways to be achieved.
Using RxJS observables, errors could be cached by the catchError operator streamed in a pipe. This operator intercepts the error from the stream and allows for custom handling. It also returns a new Observable.
Another way to do it is HTTP interceptor. This is very useful for more as a global solution.
Custom error handler is also a viable option. A custom class, provided in the AppModule for ErrorHandler.
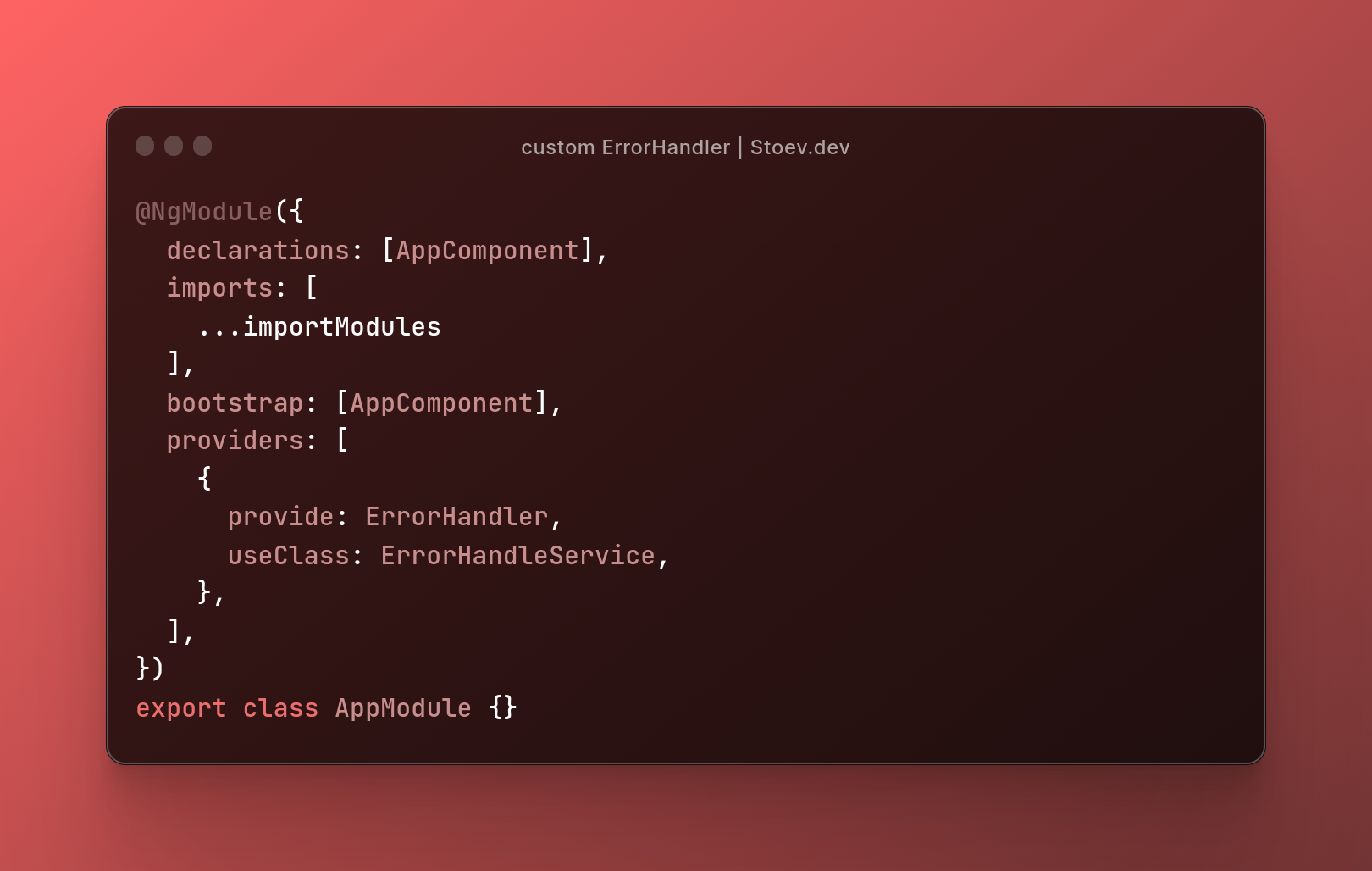
What is an Observable and Observer
I have an article on the subject with a demo project.
Check it out here for more information:
- technology
- angular
- tutorial
How I work with Observables in Angular
Answering the question.
Observables are declarative lazy push collections of multiple values. A stream of information. They could be divided into cold and hot ones.
Lazy means they emit nothing until they are subscribed!
In cold observables the data is produced inside the observable. When subscribed they emit the values, but it has to be pointed out that every subscription starts new execution!
This is called unicasting behavior.
The data is produced outside the hot observables. Since the information is not created in the observable, the data is created, even if nothing subscribes to it.
The Observer is the consumer of the value. Simply put a set of callbacks, one for each type of notification delivered by the Observable: next, error, complete
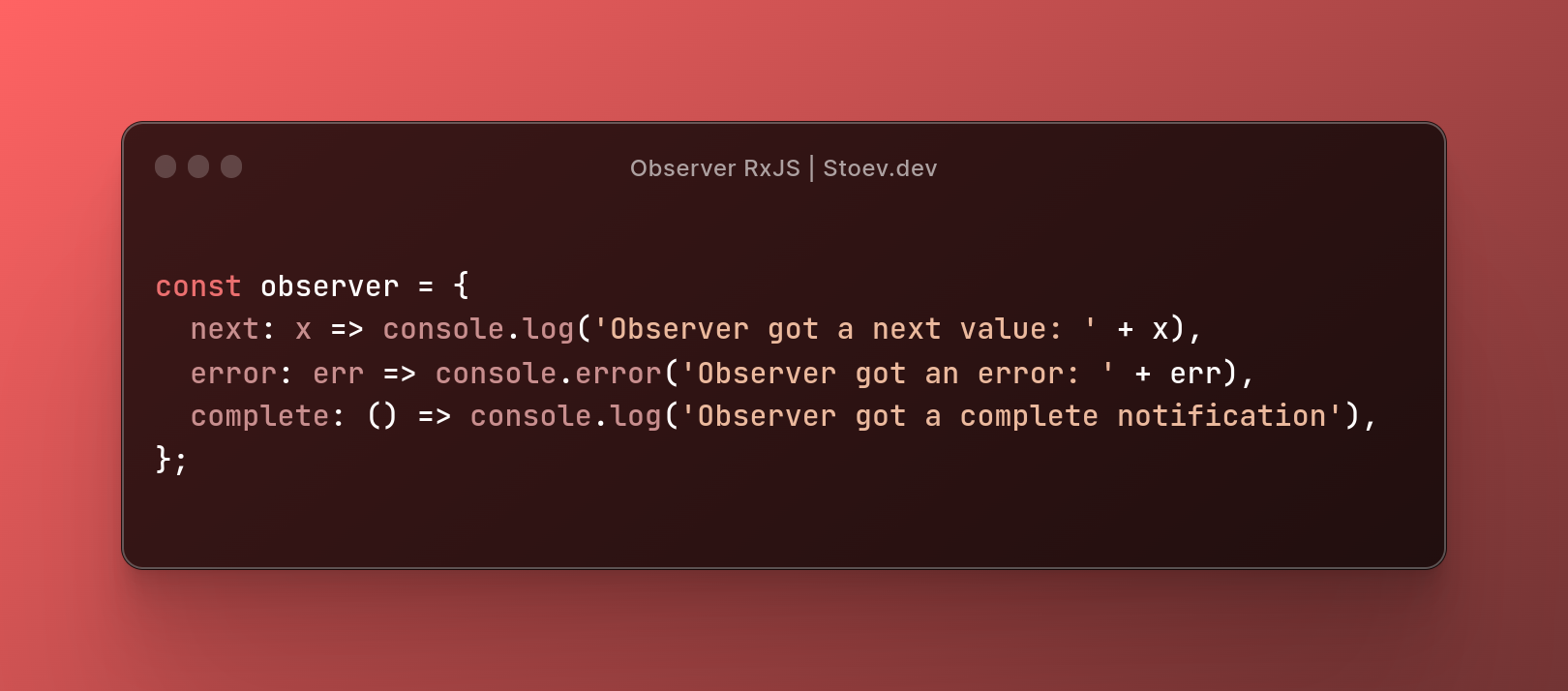
Error handling in Observables
This is very useful for catching and manipulating data in case of a problem. RxJS comes with a built in operator for that
catchError
catchError(project : function): Observable
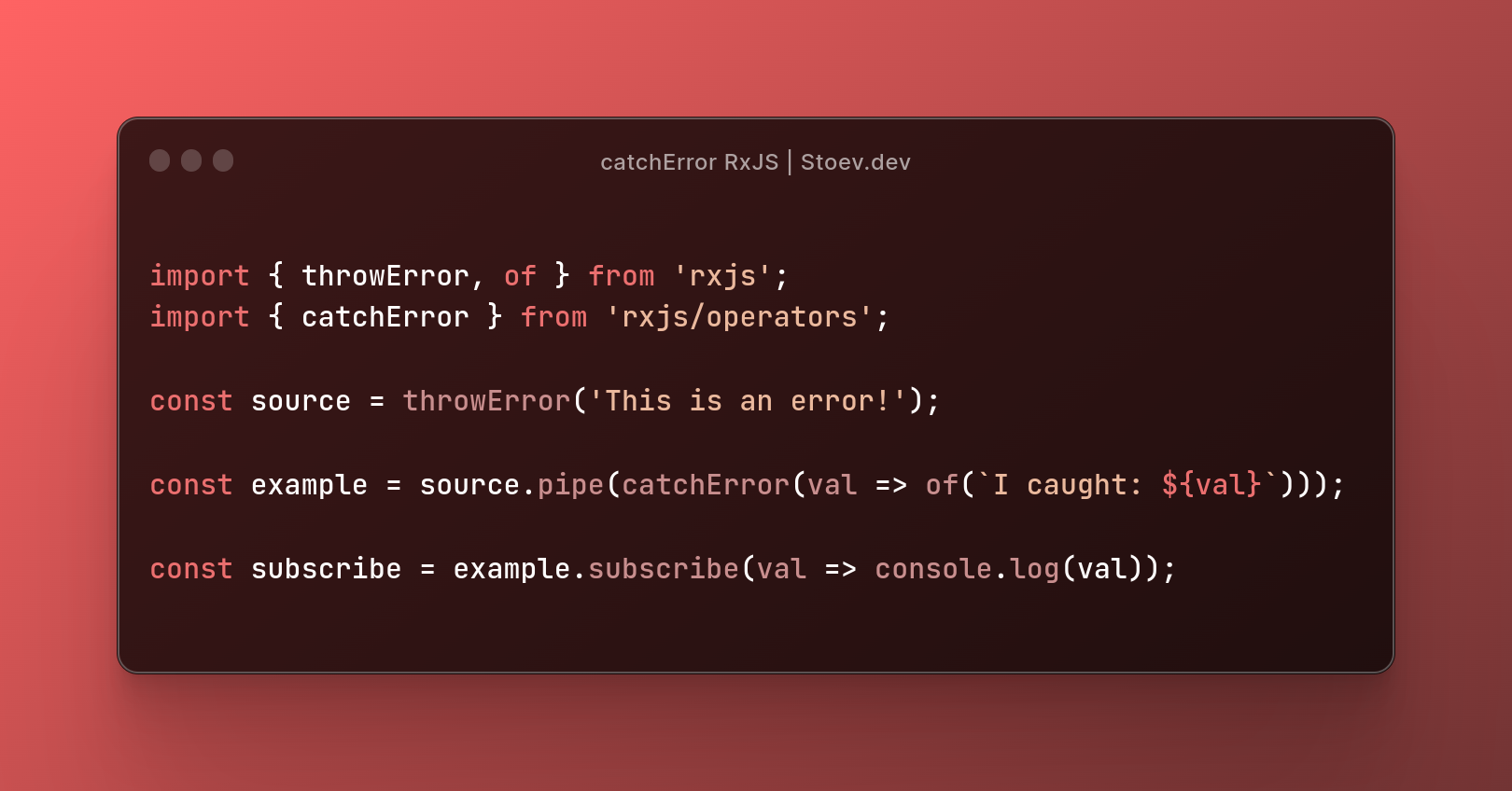
Subject, BehaviorSubject and ReplaySubject
Very powerful Observables that allows values to be multicast to many Observers.
The difference is that Observables creates new execution with every subscription, while Subject as a type of Observables shares the same value to every Observer.
Internally the Observer is registered to a list of listeners and doesn’t invoke new execution.
Every Subject is an Observer
BehaviorSubject is a variant of Subject. It stores the last value and can deliver it on demand. It represents “values over time”.
Subject doesn’t require initial value, while BehaviorSubject does!
ReplaySubject is similar to BehaviorSubject and also sends old values, but it can also record a part of the Observable execution.
Observable vs Promises
This question is tricky.
Both Promises and Observables provide us with abstractions that help us deal with the asynchronous nature of the application.
Observables are lazy and nothing is executed, until subscribed. On the other hand Promises are eager and start immediately once defined.
Promises return only one response, while Observables can emit multiple values.
Promises are executed and nothing can stop them! Observables on other hand, are cancelable.
Observables also add a lot more functionalities with RxJS operators.
What is the * in Angular directives
The asterisk is a little magic Angular adds to the pool. This convention is shorthand that Angular interprets and converts into a longer form.
It is transformed into a <ng-template> tag that surrounds the host element and its descendants.
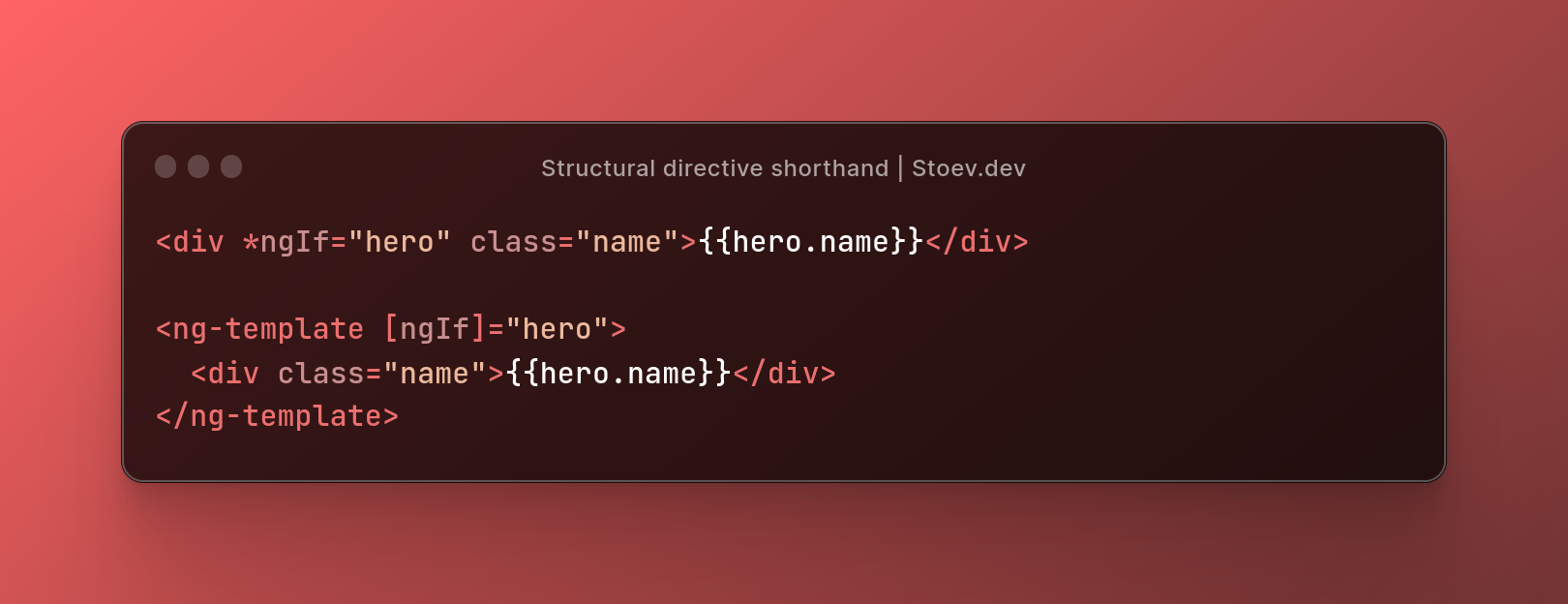
That usually leads to another question:
Types of directives
The directives in Angular are attributes that allow HTML template enhancements and add behavior to an existing DOM element.
There are:
1. Component directives - used with a template. This type of directive is the most common directive type
2. Structural directives - change the DOM layout by adding and removing DOM elements
3. Attribute directives - change the appearance or the behavior of an element, component, or another directive
ViewEncapsulation
This defines the way CSS styles are encapsulated. The types are:
1. Emulated - the default option. It adds a specific attribute to the component’s host element and applies the same attribute to all CSS selectors.
2. None - this removes all encapsulation, meaning the styles here are applicable to any HTML element of the application
3. ShadowDom - uses the browser’s native Shadow DOM API to encapsulate CSS styles
What does Shadow DOM mean?
It allows hidden DOM trees to be attached to elements in the regular DOM tree. It introduces scoped styles to the web platform.
ViewChild and ViewContent
- technology
- tutorial
- angular
Angular View Children and Content Children
ViewChild of given components is the element used within its template. It is a reference to it.
ViewContent is similar to the ViewChild, but the element is projected from the host component.
For more in-depth explanation and examples, check the article above.
What is a provider
Angular relies heavily on Dependency Injection.
Providers are instructions on how to obtain a value for a dependency.
When a service is provided to the root application injector, it’s available throughout the entire application.
It is recommended to do so!
Of course providing a service only to a specific module is possible.
When creating a service with the Angular CLI, it is provided in ‘root’.
When lazy loading a module, the services provided in the root are not the same created for the new module. Every component created with a lazy loaded module’s context, gets its own local instance of child provided services.
Reactive and Template forms
I also have an in-depth article on the subject.
- technology
- tutorial
- angular
Reactive Forms in Angular
Reactive forms could be described as a model-driven approach for creating forms in a reactive style. It follows an observable stream pattern, where form inputs and values are provided as streams of input values.
Template driven relies on directives in the template to create and manipulate the underlying object model. They are usually used for simple forms, since they are straightforward to add and implement.
Template driven forms doesn’t scale that well as Reactive forms
Lazy Loading
By default, modules are eagerly loaded in Angular. The moment the application is loaded, so do all modules.
Lazy loading is a design pattern that loads NgModules as needed. The initial bundle size is smaller.
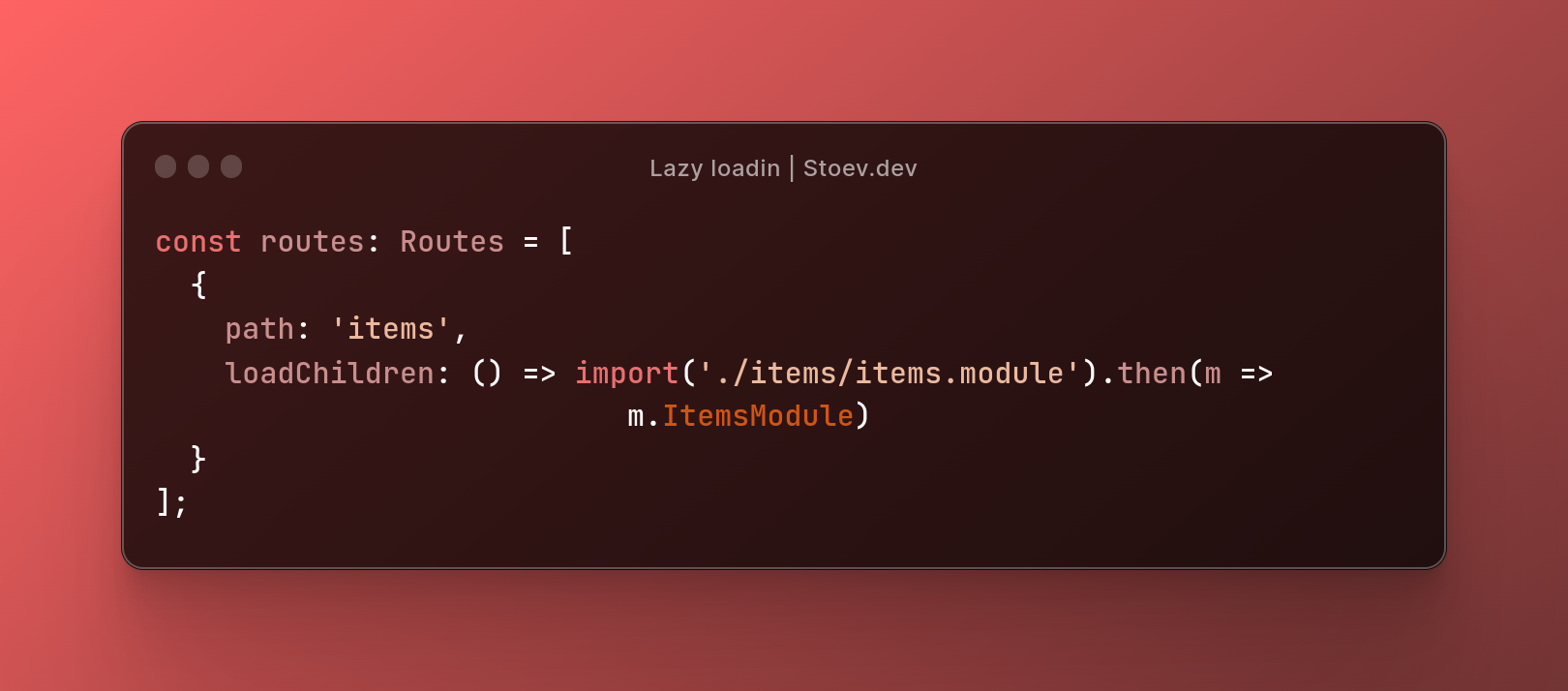
I have an article on the topic on structuring Angular applications and I briefly talked about Lazy loading and how I implement it.
Check it out here:
- tutorial
- technology
- angular
How I structure my Angular applications
HTTP Interceptors
Http Interceptors handle HttpRequests or HttpResponses
Very useful for manipulating received data.
Most interceptors also transform the outgoing request before passing it to the next interceptor in the chain.
They are usually added to the root application Injector in the AppModule.
If HttpClientModule is imported across different modules, each import creates a new copy of the HttpClientModule, which overwrites the interceptors provided in the root module.
Conclusion
All of this, as mentioned, in the previous article is nowhere complete or in-depth.
Those questions could be used as a starting point to diving into the Angular framework or as a list to remind yourself and don’t forget the technology.
Take care and happy coding!