Angular questions - PART 1
Why another list of Angular questions?
We already have like a million angular interview questions out there. Then why am I building another one?
Simple - I wanted to summarize information for me and give a starting point for more in-depth learning. So this is mostly a blueprint for what should be learned and observed!
Showing understanding is positioning yourself above the crowd.
As for the “for me” part. I am summarizing information, because I want to have some easy access to something if I need it.
With that clarified, let’s go!
Angular - how does it work
Getting most of the information here is hard, but we can point to the most important aspects.
After the Angular CLI creates a fresh angular project, we can find angular.json file in the root directory. Inside the file, a few mentions are important - “src/main.ts”, “src/index.html” and the “tsconfig.app.json” files.
The main.ts file creates a browser environment for the application.
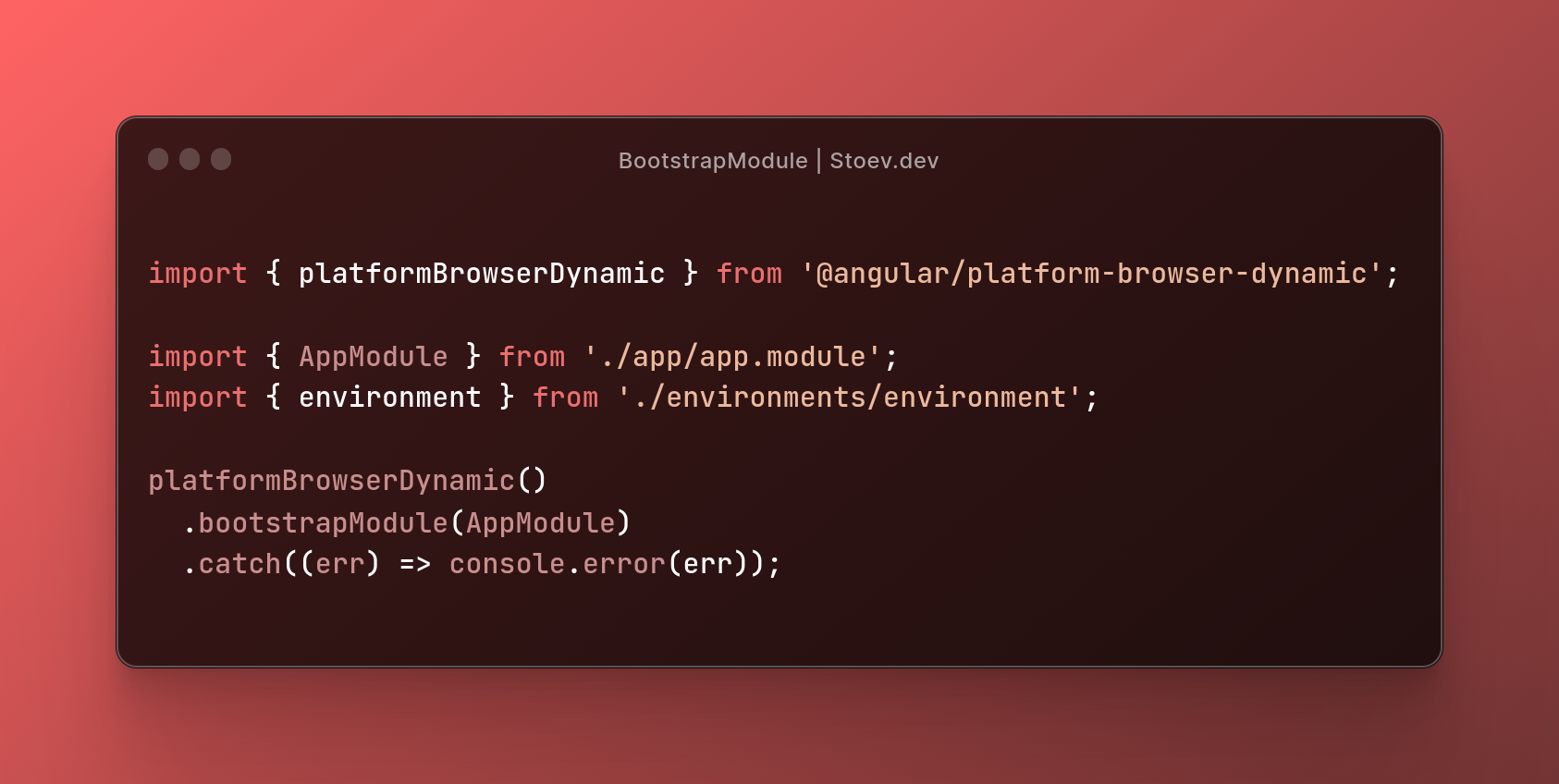
The AppModule is bootstrapped. That module itself contains all the information about the component the project requires. In that itself a few arguments could be passed. The types could be seen here
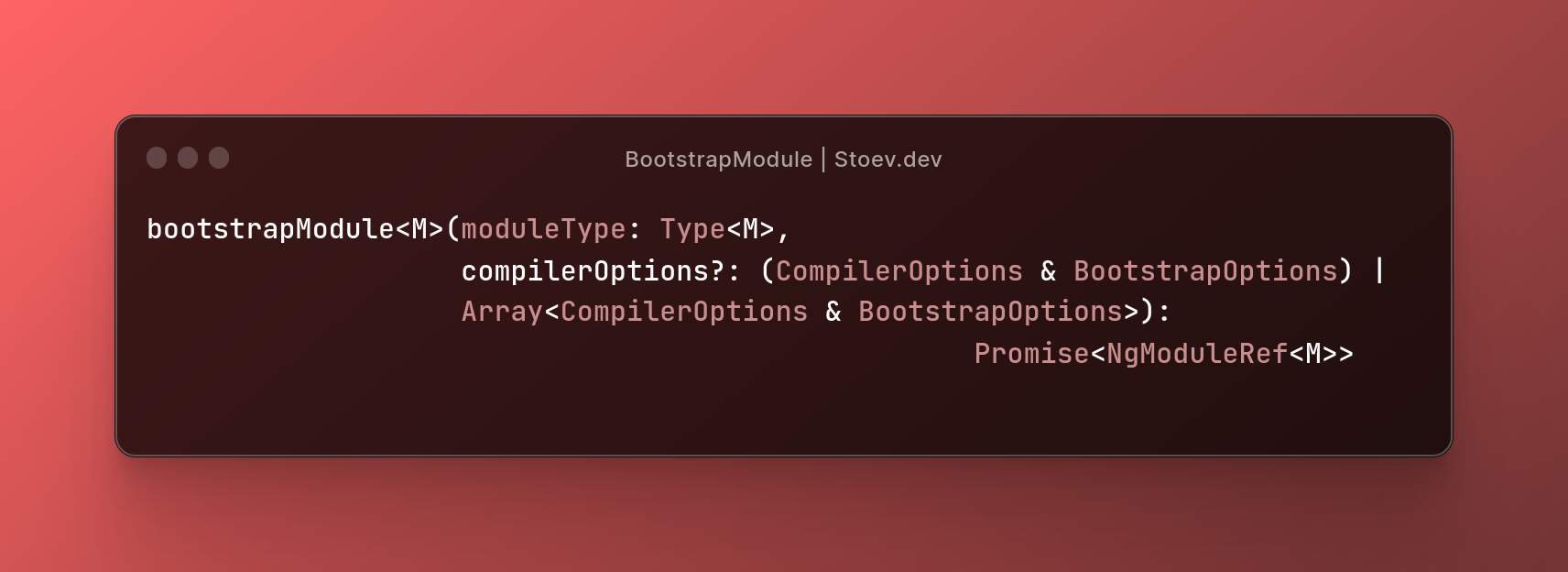
After the initialization, the project is compiled and it has the following building blocks
1. Modules
2. Components
3. Services
4. Templates
5. Directives
6. Dependency Injection
7. Metadata
I am going to explain more about these topics in the following lines and in the next two articles I have prepared.
For some ready and basic functionality Angular provides us with some built in modules, pipes and directives.
The flow is as follows. The component receives through the Injector services that it can utilize. The main TS file communicates with the HTML through Property and Event Binding.
Directives are used to enhance the HTML template. All of them require Metadata.
Angular lifecycle hooks
Knowing the lifecycle hooks is pretty essential to building performance, working and sufficient code.
We have the following in disposal:
1. ngOnChanges
2. ngOnInit
3. ngDoCheck
4. ngAfterContentInit
5. ngAfterContentChecked
6. ngAfterViewInit
7. ngAfterViewChecked
8. ngOnDestroy
ngOnChanges
This method is called when the value of a data bound property changes.
Keep in mind the way JavaScript manages objects by reference.
It’s called before the ngOnInit method.
ngOnInit
This method is called the moment Angular displays the data-bound properties. Whenever the initialization of the component is done.
This is the place you can access the information you pass with the @Input().
ngDoCheck
This method is for detection and to act on changes that Angular is not detecting on its own.
It’s called immediately after the ngOnChanges() and it runs immediately after the ngOnInit()
Avoid as much as possible, since it’s not performance friendly.
ngAfterContentInit
That method is called after the external content is loaded into the view. Or into the view that a directive is in.
It’s called once after the first ngDoCheck().
ngAfterContentChecked
Respond after Angular checks the content projected into the directive or component.
It’s called after ngAfterContentInit() and every subsequent ngDoCheck()
ngAfterViewInit()
Respond after component’s views and child views initialization.
Called once after the first ngAfterContentChecked()
ngAfterViewChecked()
Respond after component’s views and child views are checked.
Called after the ngAfterViewInit() and every subsequent ngAfterContentChecked()
ngOnDestroy()
This method is called just before Angular destroys the component or the directive.
It’s very useful for unsubscribing or managing state.
What is an Angular module?
In Angular, modules are collections of building blocks. It could include components, services, templates, directives and pipes.
It’s usually logically separated from the rest of the application and could be exported and imported.
It is structured with a metadata decorator in the following example.
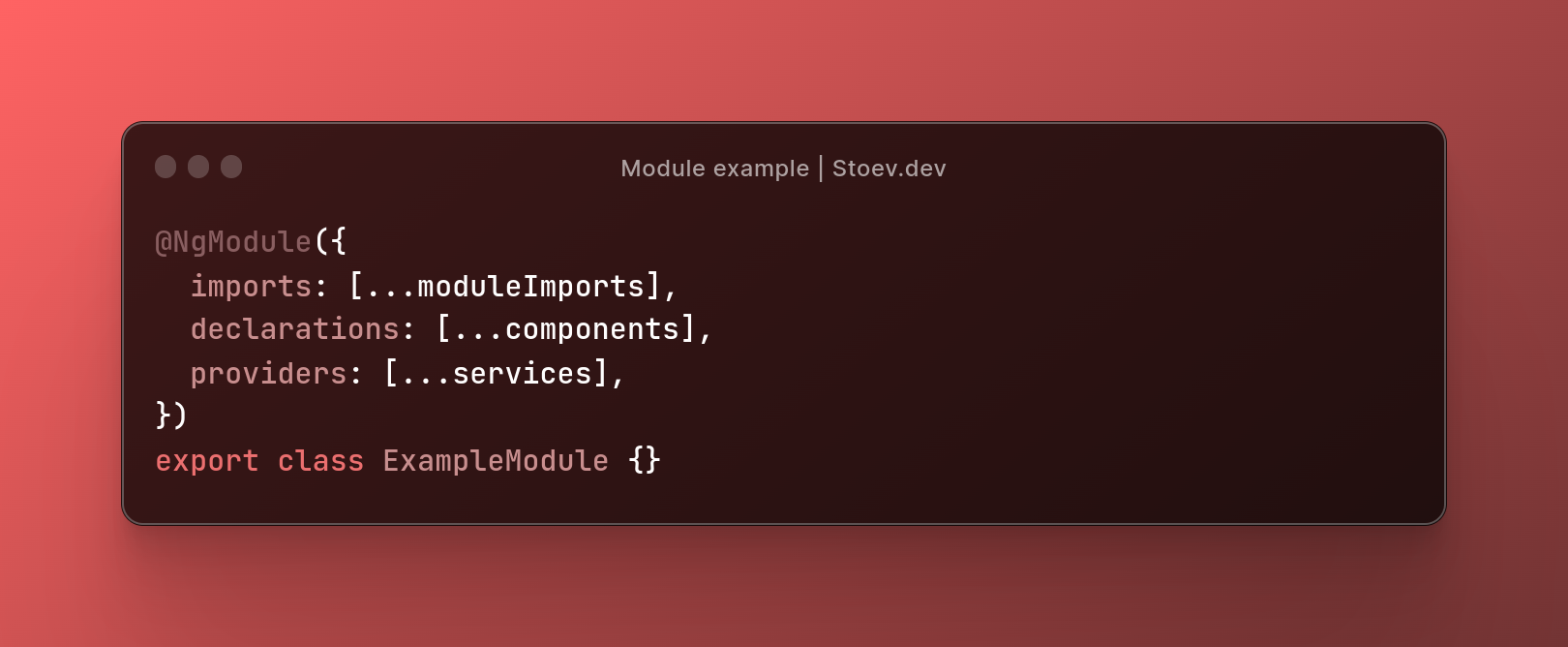
The providers option is used to configure a set of injectable services that are available in the injector of the module.
CommonModule in Angular
I have a whole article on the subject.
This is the very basic module angular has. It adds all fancy HTML attributes and directives - like *ngIf, *ngFor and etc.
- technology
- angular
- tutorial
What is CommonModule in Angular
Pipes | Pure and Impure
Short representation of “pipeline” is a function that is designed to accept an input value, transform it ( process ) and return it as an output.
Some key features:
1. It could be both pure and impure - Pure pipes use pure function. Meaning Angular calls the pipe only when it detects a change in the arguments passed. The default state of the pipe is “pure”.
Impure pipes are called with every change detection.
2. Could be chained with each other - That is one of the best features ever. The output of a pipe could be used as an Input of another pipe and so on.
3. Pipes can take as many arguments as needed.
Async pipe vs .subscribe()
This question is not really “vs” and using a manual subscription could be needed.
Most of the cases with proper observable management is not necessary and it could be used directly in the template with the | async pipe.
I have a great article on the subject!
Async pipe in general subscribes to an observable or promise and returns the latest value that is emitted.
It also marks the component to be checked for changes when a new value is emitted.
- technology
- angular
- tutorial
How I work with Observables in Angular
Key features:
1. Async pipe subscribes and unsubscribes from the observable.
2. Could be used as conditional rendering directly in the template with *ngIf directive.
3. It is associated with Declarative programming and proper Observable management.
Template expression and Template statement
Well, I have another article on the subject.
It is actually very simple.
The template expression is executed by Angular and assigned to a property that is binded in the template.
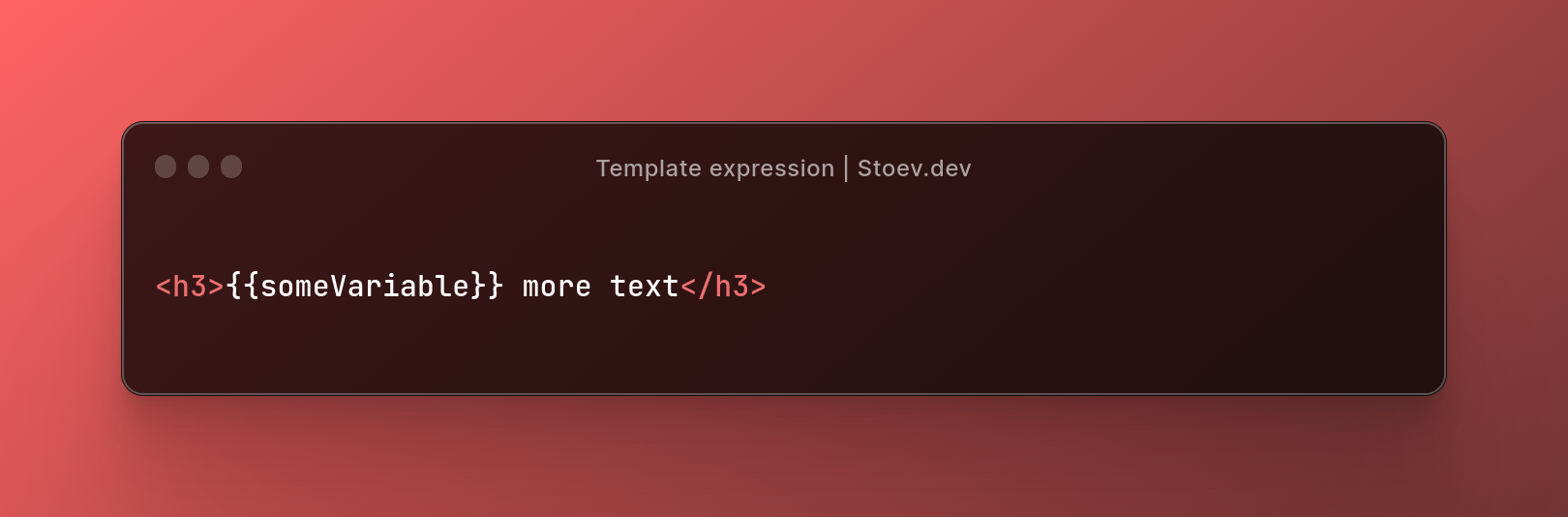
Template Statement on the other hand is reversed. It responds to an event raised by a binded target.
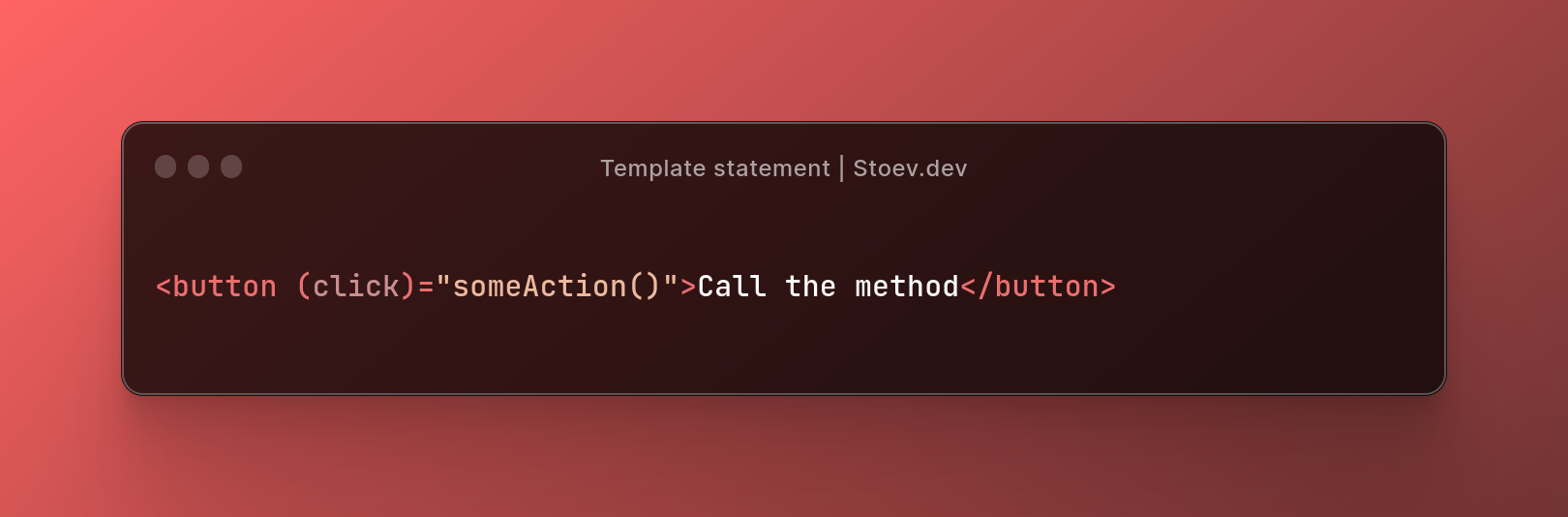
You can read more here:
- technology
- tutorial
- angular
Angular Data Binding
Dependency Injection
That is a question that requires an article on its own.
This is an important application design pattern that is not strictly connected to Angular.
It is a mechanism for creating and delivering some parts of an application to other parts of an application that requires them.
The dependencies are usually services, but could be values, such as strings or functions.
An injector for an application instantiates dependencies when needed, using a configured provider of the service or value.
Data binding
Data binding is the process and concept of communication between the template ( the DOM ) and the component.
It allows us to have dynamic and interactive applications.
There are four ways to communicate in Angular
1. Interpolation - from the component to the template.
2. Property binding - the value is passed from the component to a specially defined attribute.
While the interpolation allows only primitive data to be passed and converts it as a string, propert.
3. Events - event binding is the ability to send information back to the component from the template. It could be click, change or custom.
4. Two way data binding - Angular is awesome with that. The data could flow in both directions! From the component to the template and vice versa.
Interpolation
This is a type of data binding. In Angular it’s represented with double curly braces and converts the data to string.
Angular embeds the expressions into marked up text.
JIT vs AOT
Let’s define what JIT and AOT are.
JIT is an abbreviation of Just in time Compilation.
This means that JavaScript is compiled in the browser, during runtime. It compiles just before running and each file is compiled separately.
It's very useful and suitable for local development.
AOT on the other hand means Ahead of Time. Meaning it is compiled at build time. The code is compiled to native machine code.
It’s great because the browser downloads a pre-compiled version of the application.
It’s faster!
After Angular 9, the default is AOT.
What is zone.js
Important topic for Angular and the way it detects changes.
Zone.js is a library that serves as a signaling mechanism that captures operations like event listeners and requests.
The way this happens is Zone.js adds or changes the default behavior of elements without changing the source code. A zone aware event listener will pick the .addEventListener and will monitor it. When it is executed, a wrapper function is also executed in order for Angular to pick it up.
We have two zones in disposal here: the outer zone and the inner zone.
The outer zone is provided by Zone.js and runs outside Angular. So it won’t trigger any change detection.
The inner zone is implemented in NgZone and extends the functionality. Everything triggered here will trigger change detection.
Something to be mentioned here - we can run Angular without zone.js as a dependency, but this means we have to trigger all changes and handle them ourselves.
To remove it, remove the import in the polyfills.ts and bootstrap it with ngZone: ‘noop’
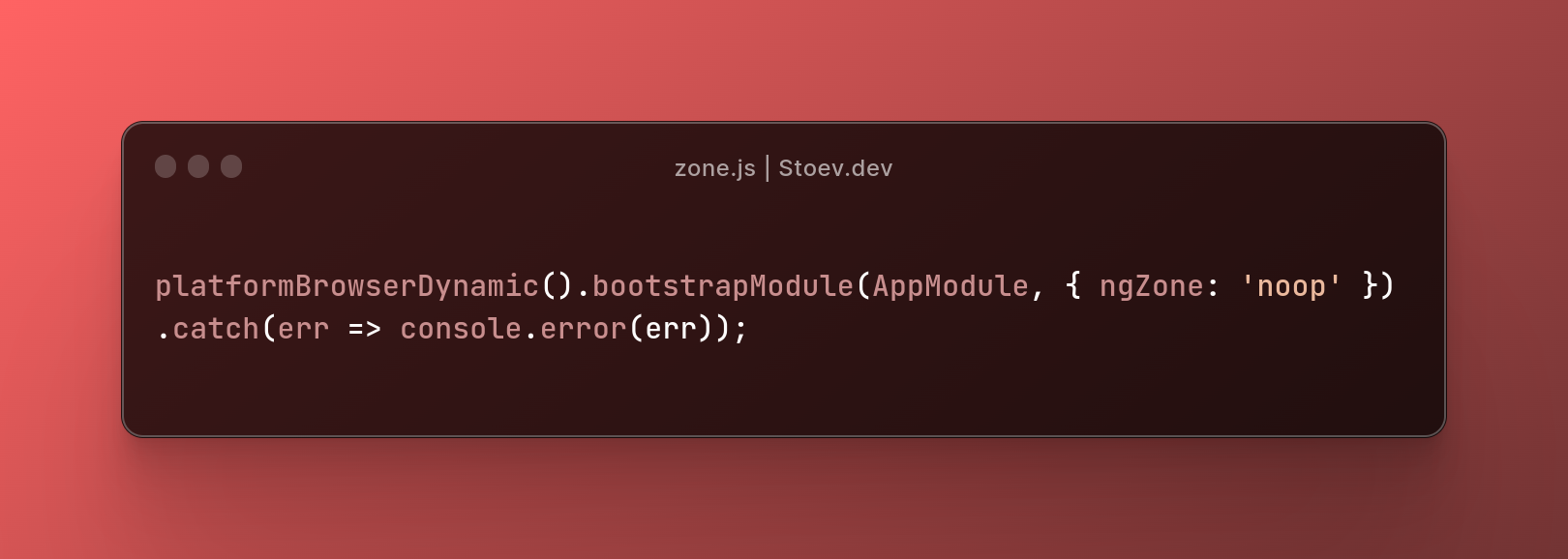
What is Angular Material
Angular Material is a library of Material Design ready to use components.
Conclusion
Everything you have read could be expanded in an article on itself. But answering Angular interview questions should be followed with examples and concepts.
When answering a question, answer from real experience.
Personal projects are an experience too.
When describing Data binding, show and explain examples that were interesting and challenging.
Never just answer the question and call it a day.
Be communicative and show you are interested in the framework.
Take care and see you in part 2!